A text layer extension for Annotorious OpenSeadragon v3. Includes support for the ALTO/XML format.
Via npm
npm install --save @annotorious/openseadragon-textlayer
Note that this extension works only with Annotorious OSD v3.
npm install --save @annotorious/openseadragon
Code example:
import { createOSDAnnotator } from '@annotorious/openseadragon';
import { mountExtension, transcriptionLabel } from '@annotorious/openseadragon-textlayer';
const viewer = OpenSeadragon({
// ...init OpenSeadragon viewer
});
// init Annotorious
const anno = createOSDAnnotator(viewer);
// init the text layer extension
const textlayer = mountExtension(anno, {
label: transcriptionLabel,
mode: 'fixedPageSize',
position: 'center',
offsetY: 60
});
// load ALTO file
textlayer.loadOCR('alto-sample.xml');
Make sure you have OpenSeadragon and the AnnotoriousOSD script and stylesheetin imported in your page.
<html>
<head>
<!-- ... -->
<!-- include script file -->
<script src="https://cdn.jsdelivr.net/npm/@annotorious/openseadragon-textlayer@latest/dist/annotorious-openseadragon-textlayer.js"></script>
<!-- include CSS styles -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@annotorious/openseadragon-textlayer@latest/dist/annotorious-openseadragon-textlayer.css">
</head>
</html>
When using script import, Annotorious and the Text Layer extension expose their APIs through
global variables AnnotoriousOSD
and OSDTextLayer
.
window.onload = function() {
var viewer = OpenSeadragon({
// ...init OpenSeadragon viewer
});
// init Annotorious
var anno = AnnotoriousOSD.createOSDAnnotator(viewer);
// init the text layer extension
var textlayer = OSDTextLayer.mountExtension(anno, {
label: OSDTextLayer.transcriptionLabel,
mode: 'fixedPageSize',
position: 'center',
offsetY: 50
});
// load ALTO file
textlayer.loadOCR('alto-sample.xml');
}
The text layer extension supports the following initialization options:
Option | Type | Default | Description |
---|---|---|---|
defaultVisible | boolean | true |
Defines whether text layer is visible by default |
label | Function | - | A function which takes an ImageAnnotation as argument and must return a string to display as label |
mode | string | fixedScreenSize |
Label display mode: fixedScreenSize , fixedPageSize or fillBounds
|
offsetX | number | 0 | X offset from the default position, pixels, original base image resolution |
offsetY | number | 0 | Y offset from the default position, pixels, original base image resolution |
position | string | bottomleft |
label position relative to the annotation bounding box: topleft , bottomleft or center
|
The textlayer
object exposes the following API methods:
-
isLocked() returns
true
if OpenSeadragon is currently locked, so mouse drag will select text -
isVisible() returns
true
if the text layer is currently visible - loadOCR(url: string) loads an ALTO/XML file from the given URL
- setLocked(locked: boolean) changes lock status of the OpenSeadragon layer
- setVisible(visible: boolean) changes the visibility of the text layer
- unmount() unmounts the extension and destroys the text layer‚
Most aspects of the appearance can be controlled via CSS. Selecting
.a9s-osd-text-layer .annotation span
allows you to override the default
styles.
.a9s-osd-textlayer .annotation span {
color: #d80bde;
font-size: 60px;
text-shadow: 0 0 6px rgb(255 255 255 / 95%);
}
This Annotorious extension exists thanks to the support of Brumfield Labs, creators of FromThePage.
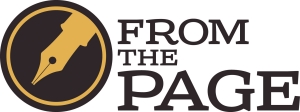
Furthermore, thanks and acknowledgements go out to Johannes Baiter whose Mirador Textoverlay plugin served as a guide for this extension.