babel-plugin-console
🎮
Adds useful build time console functions
Problem
Ever find yourself using console.log
to work out what the heck is going on within your functions?
And then put too many variables into the log and lose context of which value is which variable?
Solution
These problems can be solved by a computer at build time. This plugin allows you to inspect a functions parameters, variables, return value and parent scope by adding meaningful logs around the scope of the function - removing the hassle of manual console.log
statements or debugging.
Installation
With npm:
npm install --save-dev babel-plugin-console
With yarn:
yarn add -D babel-plugin-console
Setup
.babelrc
CLI
babel --plugins console script.js
Node
APIs
console.scope()
Outputs a collection of messages for a functions entire scope
Note: this API is the same as the standard console.log
Syntax
consolescopeobj1 obj2 ... objN;consolescopemsg subst1 ... substN;
Parameters
-
obj1 ... objN
A list of JavaScript objects to output. The string representations of each of these objects are appended together in the order listed and output. -
msg
A JavaScript string containing zero or more substitution strings. -
subst1 ... substN
JavaScript objects with which to replace substitution strings within msg. This gives you additional control over the format of the output.
Usage
Plugin
const add100 = { const oneHundred = 100; consolescope'Add 100 to another number'; return ;}; const add = { return a + b;}; ↓ ↓ ↓ ↓ ↓ ↓
Browser:
Node:
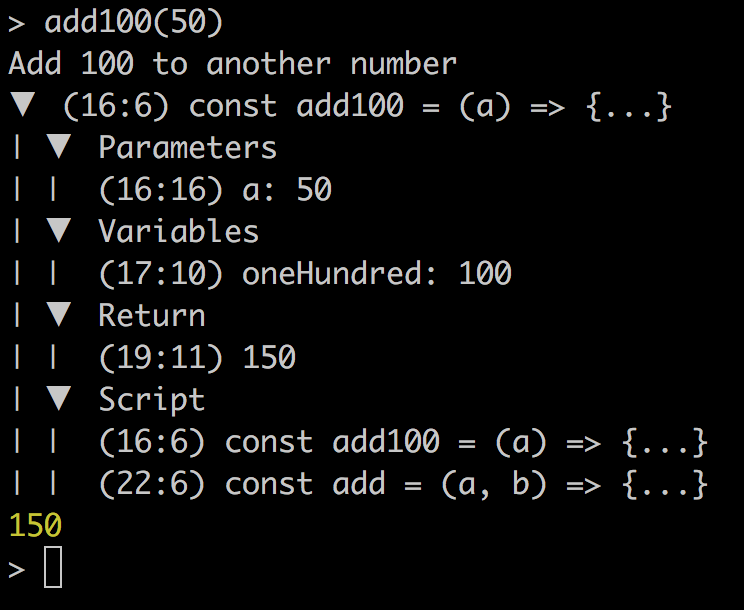
Macros
babel-plugin-console
also ships with babel-macros supported 🎉
To use a macro you will need to
install and setup babel-macros
.
Once you have enabled babel-macros
import/require the scope
macro with:
;// ORconst scope = ;
Example
Note: this is the same as the above usage and will generate the same logs - only difference is it uses the scope macro 😎
↑ ↑ ↑ ↑ ↑ ↑; const add100 = { const oneHundred = 100; ; return ;}; const add = { return a + b;};
Inspiration
This tweet led to me watching
@kentcdodds's live presentation on ASTs. This plugin is an extension on the
captains-log
demoed - Thanks Kent!
Other Solutions
You could use a debugger with breakpoints to pause the execution of a function and inspect the values of each variable.
The above add100
function would look like the following if you used a debugger with a breakpoint:
Contributors
Matt Phillips 💻 📖 🚇 ⚠️ |
Stephen Bluck 📖 |
---|
LICENSE
MIT