Pretty logger for Electron apps
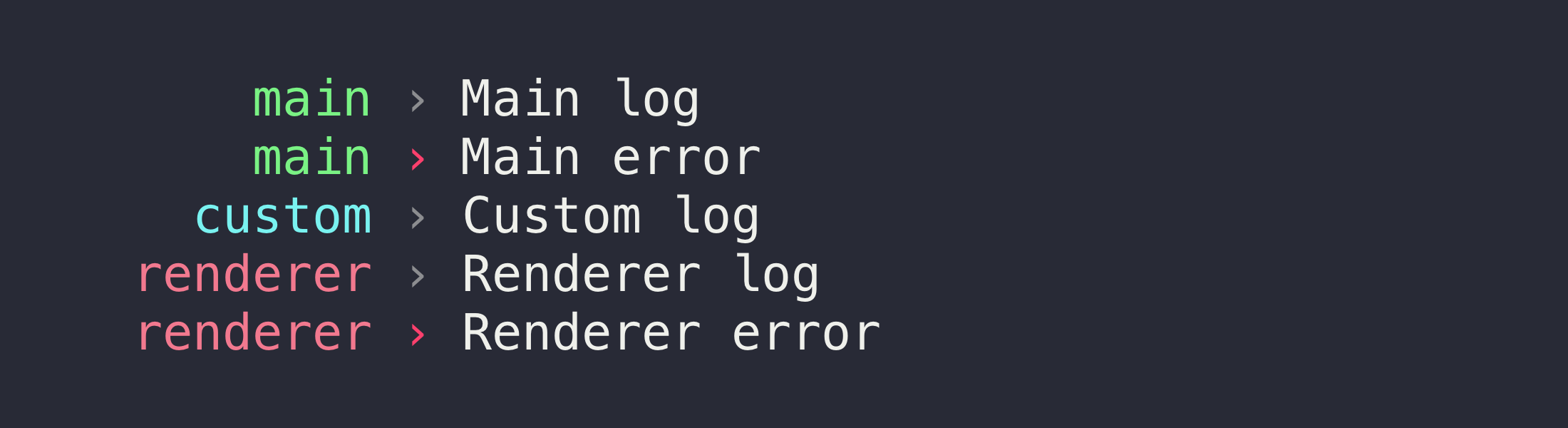
By default, logs from the renderer process don't show up in the terminal. Now they do.
You can use this module directly in both the main and renderer process.
Install
$ npm install electron-timber
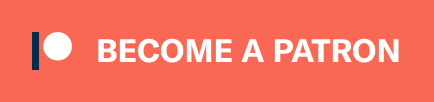
Usage
Main process:
const app BrowserWindow = ;const logger = ; let mainWindow;app;
Renderer process:
const logger = ; logger;logger;
API
logger
Logging will be prefixed with either main
or renderer
depending on where it comes from.
Logs from the renderer process only show up if you have required electron-timber
in the main process.
The methods are bound to the class instance, so you can do: const log = logger.log; log('Foo');
.
log(…values)
Like console.log
.
warn(…values)
Like console.warn
.
error(…values)
Like console.error
.
time(label)
Like console.time
.
timeEnd(label)
Like console.timeEnd
.
streamLog(stream)
Log each line in a stream.Readable
. For example, child_process.spawn(…).stdout
.
streamWarn(stream)
Same as streamLog
, but logs using console.warn
instead.
streamError(stream)
Same as streamLog
, but logs using console.error
instead.
create([options])
Create a custom logger instance.
You should initialize this on module load so prefix padding is consistent with the other loggers.
options
Type: Object
name
Type: string
Name of the logger. Used to prefix the log output. Don't use main
or renderer
.
ignore
Type RegExp
Ignore lines matching the given regex.
logLevel
Type: string
Can be info
(log everything), warn
(log warnings and errors), or error
(log errors only). Defaults to info
during development and warn
in production.
getDefaults()
Gets the default options (across main
and renderer
processes).
setDefaults([options]) Main process only
Sets the default options (across main
and renderer
processes).
options
Type: Object
Same as the options
to create()
.
Toggle loggers
You can show the output of only a subset of the loggers using the environment variable TIMBER_LOGGERS
. Here we show the output of the default renderer
logger and a custom unicorn
logger, but not the default main
logger:
TIMBER_LOGGERS=renderer,unicorn electron .
Related
- electron-util - Useful utilities for developing Electron apps and modules
- electron-reloader - Simple auto-reloading for Electron apps during development
- electron-serve - Static file serving for Electron apps
- electron-debug - Adds useful debug features to your Electron app
- electron-context-menu - Context menu for your Electron app
- electron-dl - Simplified file downloads for your Electron app
- electron-unhandled - Catch unhandled errors and promise rejections in your Electron app
Maintainers
License
MIT