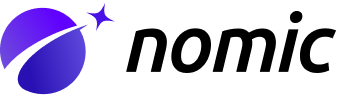
A JavaScript library for accepting Bitcoin deposits with Interchain Deposits to any EVM-based or IBC-compatible blockchain, powered by Nomic.
npm install nomic-bitcoin
import { generateDepositAddressIbc } from 'nomic-bitcoin'
let depositInfo = await generateDepositAddressIbc({
relayers: ['https://my-bitcoin-relayer.example.com:1234'],
channel: 'channel-0', // IBC channel ID on Nomic
bitcoinNetwork: 'testnet',
receiver: 'cosmos1...', // bech32 address of the depositing user
})
console.log(depositInfo)
/*
{
code: 0,
bitcoinAddress: "tb1q73yhgsjedp2uuwjew6zcj0kurryyue2zqjdgn5g5cf7w4krwgtusgsmpku",
expirationTimeMs: 1624296000000,
bridgeFeeRate: 0.015,
minerFeeRate: 0.0001,
}
*/
Bitcoin sent to bitcoinAddress
before the expiration date will be automatically IBC-transferred over the specified channel and should appear in the user's account with no further interaction required.
import { generateDepositAddressEth } from 'nomic-bitcoin'
let depositInfo = await generateDepositAddressEth({
relayers: ['https://my-bitcoin-relayer.example.com:1234'],
bitcoinNetwork: 'testnet',
ethereumNetwork: 'sepolia',
receiver: '0x...', // an Ethereum address
})
console.log(depositInfo)
/*
{
code: 0,
bitcoinAddress: "tb1q73yhgsjedp2uuwjew6zcj0kurryyue2zqjdgn5g5cf7w4krwgtusgsmpku",
expirationTimeMs: 1624296000000,
bridgeFeeRate: 0.015,
minerFeeRate: 0.0001,
}
*/
Additional Ethereum functionality including depositing to a contract call and support for other EVM chains will be released in a future upgrade.
A QR code similar to the below will be returned as a base64 data URL string and should be shown to users on desktop devices for ease of use with mobile wallets.
import { generateQRCode } from 'nomic-bitcoin'
const qrCode = await generateQRCode(depositInfo.bitcoinAddress);
The returned data URL can be used as the src
attribute on img
elements:
<img src={qrCodeData} />
The bridge currently has a capacity limit, which is the maximum amount of BTC that can be held in the bridge at any given time. When the capacity limit is reached, relayers will reject newly-broadcasted deposit addresses.
If the bridge is over capacity, the response code in depositInfo
will be 2
.
let depositInfo = await generateDepositAddressIbc(opts)
if (depositInfo.code === 2) {
console.error(`Capacity limit reached`)
}
Partner chains should communicate clearly to the user that a deposit address could not be safely generated because the bridge is currently over capacity.
When a deposit address is successfully generated, an expiration time in milliseconds is returned in depositInfo
.
let depositInfo = await generateDepositAddressIbc(opts)
if (depositInfo.code === 0) {
let { expirationTimeMs, bitcoinAddress } = depositInfo
console.log(
`Deposit address ${bitcoinAddress} expires at ${expirationTimeMs}`
)
}
[!WARNING] It is critical that the user understands that deposits to this Bitcoin address will be lost if they are sent after the expiration time. Addresses typically expire 4-5 days after creation. Do not save the address for later use, and warn the user not to reuse the address, even though multiple deposits to the same address will work as expected before the address expires.
The Nomic bridge will deduct a fee from incoming deposits. The fee rate is currently a percentage of the deposit amount, and is returned in depositInfo
.
let depositInfo = await generateDepositAddressIbc(opts)
if (depositInfo.code === 0) {
let { bridgeFeeRate, minerFeeRate, bitcoinAddress } = depositInfo
console.log(
`The fee rate for deposits to ${bitcoinAddress} is ${
bridgeFeeRate * 100
}% and ${minerFeeRate} sats per byte`
)
}
Additionally, a small fixed fee will deducted by Bitcoin miners before the deposit is processed.
These fees should be communicated clearly to the user as "Bridge fee" (a percentage) and "Bitcoin miner fee" respectively.
You can query all pending deposits by receiver address with getPendingDeposits
:
import { getPendingDeposits } from 'nomic-bitcoin'
let pendingDeposits = await getPendingDeposits(relayers, address)
console.log(pendingDeposits) // [{ confirmations: 2, txid: '...', vout: 1, amount: 100000, height: 812000 }]
Nomic uses a JSON-based destination commitment structure for incoming nBTC transfers from Ethereum or IBC chains.
For example, to withdraw nBTC directly as Bitcoin:
import { buildDestination } from 'nomic-bitcoin'
let dest = buildDestination({
bitcoinAddress: 'tb1...',
})
// Use `dest` as an ICS-20 token transfer memo,
// or argument to the Ethereum bridge contract call.
Interchain Deposits require communication with Bitcoin relayers to relay generated deposit addresses to Nomic. Where possible multiple relayers should be included, 2/3rds of the relayers must relay the generated deposit addresses for a successful deposit. Running a relayer is part of running a Nomic node, see Bitcoin Relayer for more information.
[!WARNING] The set of relayers used by your app should be selected with care. Unsucessful relaying of generated deposit addresses will result in loss of deposited funds.
- Display a deposit address QR code on desktop for mobile Bitcoin wallets.
- Display the deposit address expiration time.
- Communicate bridge and miner fees.
- Show pending deposits to users to avoid user concern during processing times.