React Native Swipe Render
An easy and simple to use React Native component that renders swipable performant pages for large lists or content. Supporting both iOS and Android. Check out the docs.
- Supports smart or minimal rendering for large lists.
- Supports two ways of rendering contents. Render using function and data or render React.Element children.
- Horizontal and vertical paging for both Android and iOS.
- Initial index can be placed anywhere. Supporting both Android and iOS.
- Dynamic index support for iOS.
- Optional slide looping.
- Optional automatic slides.
- Optional alternative usage with Android
ScrollView
instead ofViewPagerAndroid
. - Supports both iOS and Android.
🔗 Quick Links
💎 Install
Type in the following to the command line to install the module.
$ npm install --save react-native-swipe-render
or
$ yarn add react-native-swipe-render
🎉 Usage Example One
Add an import
to the top of the file. At minimal, place array
data into the data
prop and render the pages using the renderItem
prop.
If you like
react-native-swipe-render
, please be sure to give it a star at GitHub. Thanks.
;; //... { return <SwipeRender data= uri: "https://luehangs.site/pic-chat-app-images/pexels-photo-853168.jpeg" uri: "https://luehangs.site/pic-chat-app-images/animals-avian-beach-760984.jpg" uri: "https://luehangs.site/pic-chat-app-images/beautiful-beautiful-woman-beauty-9763.jpg" uri: "https://luehangs.site/pic-chat-app-images/photo-755745.jpeg" uri: "https://luehangs.site/pic-chat-app-images/photo-799443.jpeg" renderItem= { return <View key="SwipeRender-slide#" + index style=flex: 1 backgroundColor: "#000"> <Image source= uri: itemuri style=flex: 1 resizeMode="contain" /> </View> ; } // OPTIONAL PROP USAGE. index=0 // default 0 loop=false // default false loadMinimal=true // default false loadMinimalSize=2 horizontal=true // default true enableAndroidViewPager=false // default ScrollView // TO ENABLE AndroidViewPager: // react-native >= 0.60 - install @react-native-community/viewpager separately // react-native < 0.60 - ready to go! /> ;}//...
🎉 Usage Example Two
Add an import
to the top of the file. At minimal, wrap any view in the <SwipeRender></SwipeRender>
.
If you like
react-native-swipe-render
, please be sure to give it a star at GitHub. Thanks.
;; //... { return <SwipeRender // OPTIONAL PROP USAGE. index=0 // default 0 loop=false // default false loadMinimal=true // default false loadMinimalSize=2 horizontal=true // default true enableAndroidViewPager=false // default ScrollView // TO ENABLE AndroidViewPager: // react-native >= 0.60 - install @react-native-community/viewpager separately // react-native < 0.60 - ready to go! > <View style=flex: 1 backgroundColor: "#000"> <Image source= uri: "https://luehangs.site/pic-chat-app-images/pexels-photo-853168.jpeg" style=flex: 1 resizeMode="contain" /> </View> <View style=flex: 1 justifyContent: "center" alignItems: "center"> <Text style=color: "blue" fontSize: 25 fontWeight: "bold"> Any kind of View </Text> </View> <View style=flex: 1 backgroundColor: "#000"> <Image source= uri: "https://luehangs.site/pic-chat-app-images/beautiful-beautiful-woman-beauty-9763.jpg" style=flex: 1 resizeMode="contain" /> </View> </SwipeRender> ;}//...
⌚️ Performance Optimization List Example
If you like
react-native-swipe-render
, please be sure to give it a star at GitHub. Thanks.
;; //... { return <SwipeRender data= uri: "https://luehangs.site/pic-chat-app-images/pexels-photo-853168.jpeg" uri: "https://luehangs.site/pic-chat-app-images/animals-avian-beach-760984.jpg" uri: "https://luehangs.site/pic-chat-app-images/beautiful-beautiful-woman-beauty-9763.jpg" uri: "https://luehangs.site/pic-chat-app-images/photo-755745.jpeg" uri: "https://luehangs.site/pic-chat-app-images/photo-799443.jpeg" // Test with 100s to 1000s of data to be rendered // ... // ... // ... renderItem= { return <View key=index style=flex: 1 backgroundColor: "#000"> <Image source= uri: itemuri style=flex: 1 resizeMode="contain" /> </View> ; } index=3 // Initial index can be placed anywhere. Dynamic index support for only iOS. loadMinimal=true loadMinimalSize=2 removeClippedSubviews=true /> ;}//...
📖 Full Documentation
Learn more about the installation and how to use this package in the updated documentation page.
👏 Contribute
Pull requests are welcomed.
🎩 Contributors
Contributors will be posted here.
👶 Beginners
Not sure where to start, or a beginner? Take a look at the issues page.
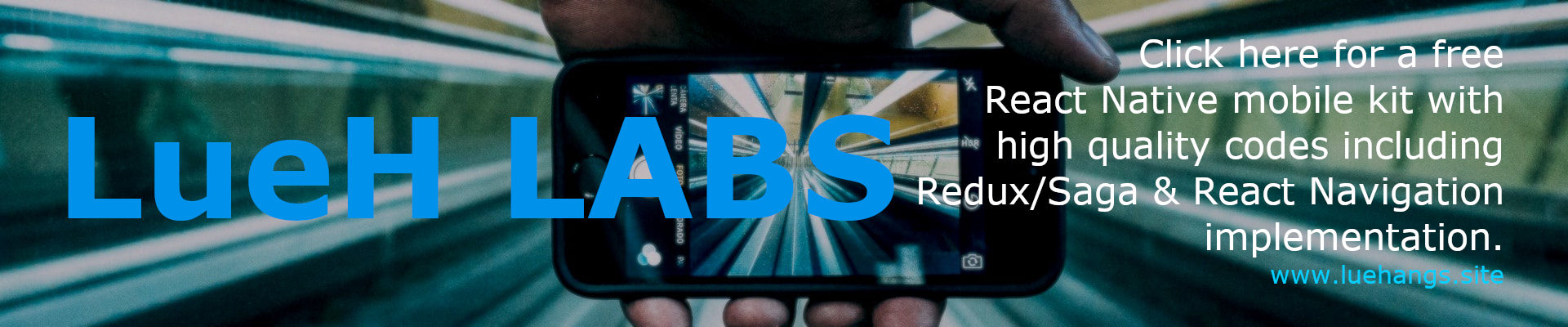
📄 License
MIT © Lue Hang, as found in the LICENSE file.