Simple Org Chart for React
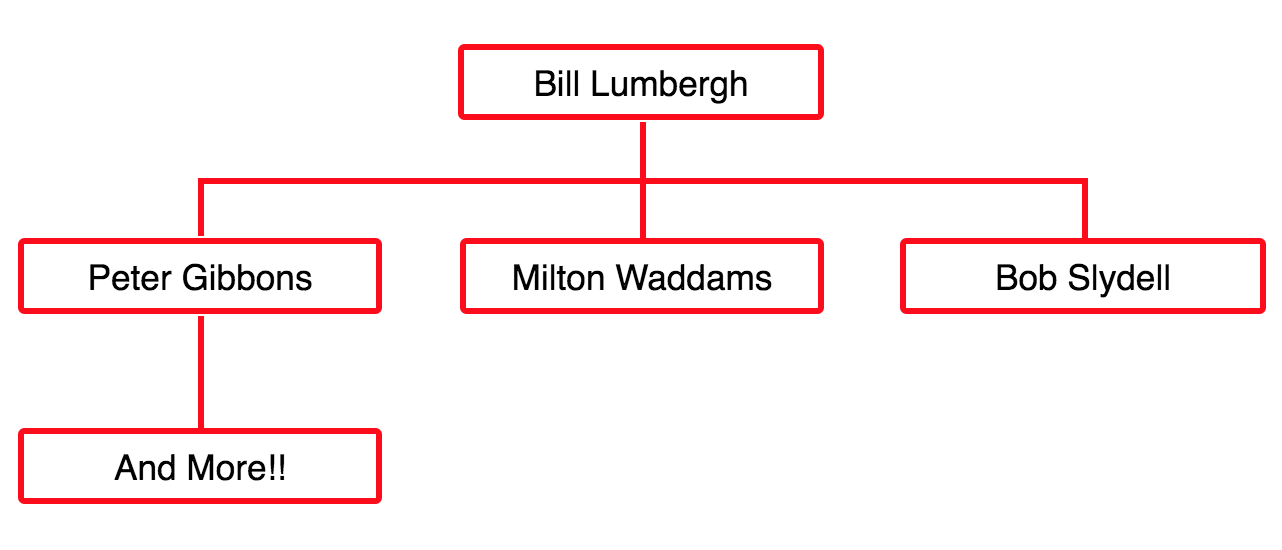
See the example project for demonstration of creating org chart data structure and using the OrgChart component.
This project focuses on the simplicity of its api.
Installation: Import Javascript and CSS
import OrgChart from 'react-orgchart';import 'react-orgchart/index.css';
Step 1: Create the org chart tree as an object literal.
The children
property of each node are rendered as children nodes.
const initechOrg = name: "Bill Lumbergh" actor: "Gary Cole" children: name: "Peter Gibbons" actor: "Ron Livingston" children: name: "And More!!" actor: "This is just to show how to build a complex tree with multiple levels of children. Enjoy!" name: "Milton Waddams" actor: "Stephen Root" name: "Bob Slydell" actor: "John C. McGi..." ;
Step 2: Define a React Component for the nodes which receives each node object literal as a prop.
You can easily add functionality as you see fit to this node component. Pass down necessary data through the tree structure outlined above.
const MyNodeComponent = node return <div ="initechNode" => nodename </div> ;;
OrgChart
component to your app.
Final Step: Add the <OrgChart = = />
Additionally, you may want to style your org chart.
See Example project stylesheet for ideas.
Customizing Styles
Simple wrap your org chart in a div with an id and define styles like this: