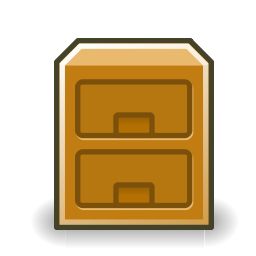
This Webpack plugin allows you to copy, archive (.zip/.tar/.tar.gz), move, delete files and directories before and after builds
npm install filemanager-webpack-plugin --save-dev
# or
yarn add filemanager-webpack-plugin --dev
// webpack.config.js:
const FileManagerPlugin = require('filemanager-webpack-plugin');
export default {
// ...rest of the config
plugins: [
new FileManagerPlugin({
events: {
onEnd: {
copy: [
{ source: '/path/fromfile.txt', destination: '/path/tofile.txt' },
{ source: '/path/**/*.js', destination: '/path' },
],
move: [
{ source: '/path/from', destination: '/path/to' },
{ source: '/path/fromfile.txt', destination: '/path/tofile.txt' },
],
delete: ['/path/to/file.txt', '/path/to/directory/'],
mkdir: ['/path/to/directory/', '/another/directory/'],
archive: [
{ source: '/path/from', destination: '/path/to.zip' },
{ source: '/path/**/*.js', destination: '/path/to.zip' },
{ source: '/path/fromfile.txt', destination: '/path/to.zip' },
{ source: '/path/fromfile.txt', destination: '/path/to.zip', format: 'tar' },
{
source: '/path/fromfile.txt',
destination: '/path/to.tar.gz',
format: 'tar',
options: {
gzip: true,
gzipOptions: {
level: 1,
},
globOptions: {
// https://github.com/Yqnn/node-readdir-glob#options
dot: true,
},
},
},
],
},
},
}),
],
};
new FileManagerPlugin({
events: {
onStart: {},
onEnd: {},
},
runTasksInSeries: false,
runOnceInWatchMode: false,
});
-
onStart
: Commands to execute before Webpack begins the bundling process
Note:
OnStart might execute twice for file changes in webpack context.
new webpack.WatchIgnorePlugin({
paths: [/copied-directory/],
});
-
onEnd
: Commands to execute after Webpack has finished the bundling process
Copy individual files or entire directories from a source folder to a destination folder. Also supports glob pattern.
[
{ source: '/path/from', destination: '/path/to' },
{
source: '/path/**/*.js',
destination: '/path',
options: {
flat: false,
preserveTimestamps: true,
overwrite: true,
},
globOptions: {
dot: true,
},
},
{ source: '/path/fromfile.txt', destination: '/path/tofile.txt' },
{ source: '/path/**/*.{html,js}', destination: '/path/to' },
{ source: '/path/{file1,file2}.js', destination: '/path/to' },
];
Options
- source[
string
] - a file or a directory or a glob - destination[
string
] - a file or a directory. - options [
object
] - copy options - globOptions [
object
] - options to forward to glob options(See available options here)
[!NOTE]
- if source is a
glob
, destination must be a directory- if source is a
file
and destination is a directory, the file will be copied into the directory
Delete individual files or entire directories. Also supports glob pattern
['/path/to/file.txt', '/path/to/directory/', '/another-path/to/directory/**.js'];
or
[
{
source: '/path/to/file.txt',
options: {
force: true,
},
},
];
Move individual files or entire directories.
[
{ source: '/path/from', destination: '/path/to' },
{ source: '/path/fromfile.txt', destination: '/path/tofile.txt' },
];
Options
- source[
string
] - a file or a directory or a glob - destination[
string
] - a file or a directory.
Create a directory path with given path
['/path/to/directory/', '/another/directory/'];
Archive individual files or entire directories. Defaults to .zip unless 'format' and 'options' provided. Uses node-archiver
[
{ source: '/path/from', destination: '/path/to.zip' },
{ source: '/path/**/*.js', destination: '/path/to.zip' },
{ source: '/path/fromfile.txt', destination: '/path/to.zip' },
{ source: '/path/fromfile.txt', destination: '/path/to.zip', format: 'tar' },
{
source: '/path/fromfile.txt',
destination: '/path/to.tar.gz',
format: 'tar', // optional
options: {
// see https://www.archiverjs.com/docs/archiver
gzip: true,
gzipOptions: {
level: 1,
},
globOptions: {
// https://github.com/Yqnn/node-readdir-glob#options
dot: true,
},
},
},
];
- source[
string
] - a file or a directory or a glob - destination[
string
] - a file. - format[
string
] - Optional. Defaults to extension in destination filename. - options[
object
] - Refer https://www.archiverjs.com/archiver
If you need to preserve the order in which operations will run you can set the onStart and onEnd events to be Arrays. In this example below, in the onEnd event the copy action will run first, and then the delete after:
{
onEnd: [
{
copy: [{ source: './dist/bundle.js', destination: './newfile.js' }],
},
{
delete: ['./dist/bundle.js'],
},
];
}
-
runTasksInSeries [
boolean
] - Run tasks in series. Defaults to false -
runOnceInWatchMode [
boolean
] - TheonStart
event will be run only once in watch mode. Defaults to false
For Example, the following will run one after the other
copy: [
{ source: 'dist/index.html', destination: 'dir1/' },
{ source: 'dir1/index.html', destination: 'dir2/' },
];
-
context [
string
] - The directory, an absolute path, for resolving files. Defaults to webpack context.