The @socket.io/redis-adapter
package allows broadcasting packets between multiple Socket.IO servers.
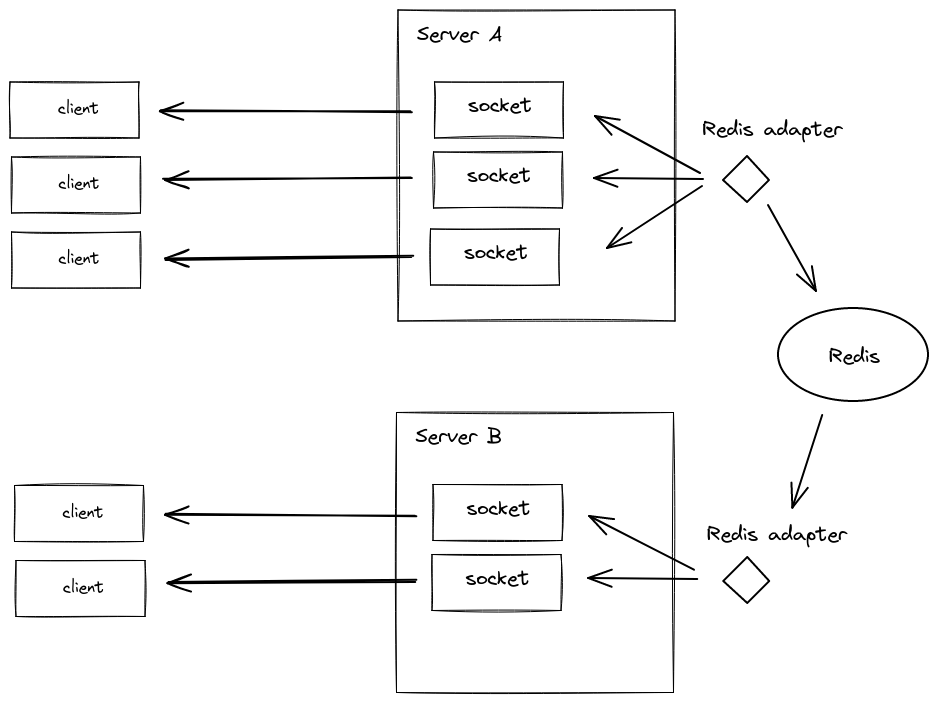
Table of contents
Feature |
socket.io version |
Support |
---|---|---|
Socket management | 4.0.0 |
✅ YES (since version 6.1.0 ) |
Inter-server communication | 4.1.0 |
✅ YES (since version 7.0.0 ) |
Broadcast with acknowledgements | 4.5.0 |
✅ YES (since version 7.2.0 ) |
Connection state recovery | 4.6.0 |
❌ NO |
npm install @socket.io/redis-adapter
Redis Adapter version | Socket.IO server version |
---|---|
4.x | 1.x |
5.x | 2.x |
6.0.x | 3.x |
6.1.x | 4.x |
7.x and above | 4.3.1 and above |
import { createClient } from "redis";
import { Server } from "socket.io";
import { createAdapter } from "@socket.io/redis-adapter";
const pubClient = createClient({ url: "redis://localhost:6379" });
const subClient = pubClient.duplicate();
await Promise.all([
pubClient.connect(),
subClient.connect()
]);
const io = new Server({
adapter: createAdapter(pubClient, subClient)
});
io.listen(3000);
import { createCluster } from "redis";
import { Server } from "socket.io";
import { createAdapter } from "@socket.io/redis-adapter";
const pubClient = createCluster({
rootNodes: [
{
url: "redis://localhost:7000",
},
{
url: "redis://localhost:7001",
},
{
url: "redis://localhost:7002",
},
],
});
const subClient = pubClient.duplicate();
await Promise.all([
pubClient.connect(),
subClient.connect()
]);
const io = new Server({
adapter: createAdapter(pubClient, subClient)
});
io.listen(3000);
import { Redis } from "ioredis";
import { Server } from "socket.io";
import { createAdapter } from "@socket.io/redis-adapter";
const pubClient = new Redis();
const subClient = pubClient.duplicate();
const io = new Server({
adapter: createAdapter(pubClient, subClient)
});
io.listen(3000);
import { Cluster } from "ioredis";
import { Server } from "socket.io";
import { createAdapter } from "@socket.io/redis-adapter";
const pubClient = new Cluster([
{
host: "localhost",
port: 7000,
},
{
host: "localhost",
port: 7001,
},
{
host: "localhost",
port: 7002,
},
]);
const subClient = pubClient.duplicate();
const io = new Server({
adapter: createAdapter(pubClient, subClient)
});
io.listen(3000);
Sharded Pub/Sub was introduced in Redis 7.0 in order to help scaling the usage of Pub/Sub in cluster mode.
Reference: https://redis.io/docs/interact/pubsub/#sharded-pubsub
A dedicated adapter can be created with the createShardedAdapter()
method:
import { Server } from "socket.io";
import { createClient } from "redis";
import { createShardedAdapter } from "@socket.io/redis-adapter";
const pubClient = createClient({ host: "localhost", port: 6379 });
const subClient = pubClient.duplicate();
await Promise.all([
pubClient.connect(),
subClient.connect()
]);
const io = new Server({
adapter: createShardedAdapter(pubClient, subClient)
});
io.listen(3000);
Minimum requirements:
- Redis 7.0
redis@4.6.0
Note: it is not currently possible to use the sharded adapter with the ioredis
package and a Redis cluster (reference).
Name | Description | Default value |
---|---|---|
key |
The prefix for the Redis Pub/Sub channels. | socket.io |
requestsTimeout |
After this timeout the adapter will stop waiting from responses to request. | 5_000 |
publishOnSpecificResponseChannel |
Whether to publish a response to the channel specific to the requesting node. | false |
parser |
The parser to use for encoding and decoding messages sent to Redis. | - |
Name | Description | Default value |
---|---|---|
channelPrefix |
The prefix for the Redis Pub/Sub channels. | socket.io |
subscriptionMode |
The subscription mode impacts the number of Redis Pub/Sub channels used by the adapter. | dynamic |