
Provides synchronous functions to read/write HLS playlists (conforms to the HLS spec rev.23, the Apple Low-Latency Spec rev. 2020/02/05, and HLS.js's Low-Latency spec)

import { parse, types, stringify } from 'hls-parser';
// Parse the playlist
const playlist = parse(textData);
// You can access the playlist as a JS object
if (playlist.isMasterPlaylist) {
// Master playlist
} else {
// Media playlist
}
// Create a new playlist
const {MediaPlaylist, Segment} = types;
const obj = new MediaPlaylist({
targetDuration: 9,
playlistType: 'VOD',
segments: [
new Segment({
uri: 'low/1.m3u8',
duration: 9
})
]
});
// Convert the object into a text
stringify(obj);
/*
#EXTM3U
#EXT-X-TARGETDURATION:9
#EXT-X-PLAYLIST-TYPE:VOD
#EXTINF:9,
low/1.m3u8
*/
Converts a text playlist into a structured JS object
Name |
Type |
Required |
Default |
Description |
str |
string |
Yes |
N/A |
A text data that conforms to the HLS playlist spec
|
An instance of either MasterPlaylist
or MediaPlaylist
(See Data format below.)
HLS.stringify(obj, postProcess)
Converts a JS object into a plain text playlist
Name |
Type |
Required |
Default |
Description |
obj |
MasterPlaylist or MediaPlaylist (See Data format below.) |
Yes |
N/A |
An object returned by HLS.parse() or a manually created object |
postProcess |
PostProcess |
No |
undefined |
A function to be called for each segment or variant to manipulate the output. |
Property |
Type |
Required |
Default |
Description |
segmentProcessor |
(lines: string[], start: number, end: number, segment: Segment, i: number) => void |
No |
undefined |
A function to manipulate the segment output. |
variantProcessor |
(lines: string[], start: number, end: number, variant: Variant, i: number) => void |
No |
undefined |
A function to manipulate the variant output. |
A text data that conforms to the HLS playlist spec
Updates the option values
Name |
Type |
Required |
Default |
Description |
obj |
Object |
Yes |
{} |
An object holding option values which will be used to overwrite the internal option values. |
Name |
Type |
Default |
Description |
strictMode |
boolean |
false |
If true, the function throws an error when parse /stringify failed. If false, the function just logs the error and continues to run. |
allowClosedCaptionsNone |
boolean |
false |
If true, CLOSED-CAPTIONS attribute on the EXT-X-STREAM-INF tag will be set to the enumerated-string value NONE when there are no closed-captions. See CLOSED-CAPTIONS
|
silent |
boolean |
false |
If true, console.error will be suppressed. |
Retrieves the current option values
A cloned object containing the current option values
An object that holds all the classes described below.
This section describes the structure of the object returned by parse()
method.
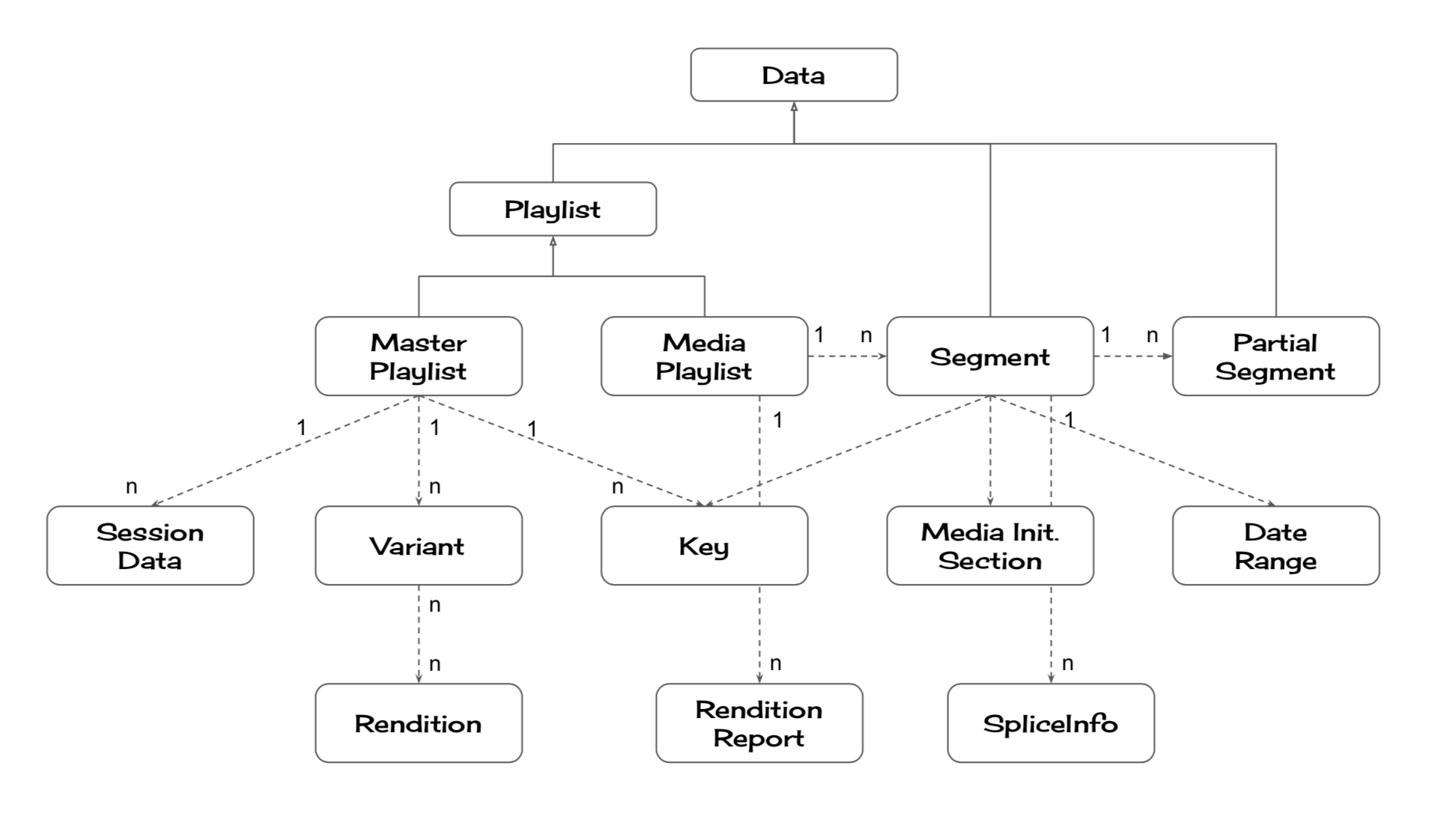
Property |
Type |
Required |
Default |
Description |
type |
string |
Yes |
N/A |
Either playlist or segment or part } |
Property |
Type |
Required |
Default |
Description |
isMasterPlaylist |
boolean |
Yes |
N/A |
true if this playlist is a master playlist |
uri |
string |
No |
undefined |
Playlist URL |
version |
number |
No |
undefined |
See EXT-X-VERSION
|
independentSegments |
boolean |
No |
false |
See EXT-X-INDEPENDENT-SEGMENTS
|
start |
object({offset: number, precise: boolean}) |
No |
undefined |
See EXT-X-START
|
source |
string |
No |
undefined |
The unprocessed text of the playlist |
MasterPlaylist
(extends Playlist
)
Property |
Type |
Required |
Default |
Description |
uri |
string |
Yes |
N/A |
URI of the variant playlist |
isIFrameOnly |
boolean |
No |
undefined |
true if the variant is an I-frame media playlist. See EXT-X-I-FRAME-STREAM-INF
|
bandwidth |
number |
Yes |
N/A |
See BANDWIDTH attribute in EXT-X-STREAM-INF
|
averageBandwidth |
number |
No |
undefined |
See AVERAGE-BANDWIDTH attribute in EXT-X-STREAM-INF
|
score |
number |
No |
undefined |
See SCORE attribute in EXT-X-STREAM-INF
|
codecs |
string |
No |
undefined |
See CODECS attribute in EXT-X-STREAM-INF
|
resolution |
object ({width: number, height: number}) |
No |
undefined |
See RESOLUTION attribute in EXT-X-STREAM-INF
|
frameRate |
number |
No |
undefined |
See FRAME-RATE attribute in EXT-X-STREAM-INF
|
hdcpLevel |
string |
No |
undefined |
See HDCP-LEVEL attribute in EXT-X-STREAM-INF
|
allowedCpc |
[object ({format: string, cpcList: [string]})] |
No |
undefined |
See ALLOWED-CPC attribute in EXT-X-STREAM-INF
|
videoRange |
string {"SDR","HLG","PQ"} |
No |
undefined |
See VIDEO-RANGE attribute in EXT-X-STREAM-INF
|
stableVariantId |
string |
No |
undefined |
See STABLE-VARIANT-ID attribute in EXT-X-STREAM-INF
|
audio |
[Rendition (type='AUDIO')] |
No |
[] |
See AUDIO attribute in EXT-X-STREAM-INF
|
video |
[Rendition (type='VIDEO')] |
No |
[] |
See VIDEO attribute in EXT-X-STREAM-INF
|
subtitles |
[Rendition (type='SUBTITLES')] |
No |
[] |
See SUBTITLES attribute in EXT-X-STREAM-INF
|
closedCaptions |
[Rendition (type='CLOSED-CAPTIONS')] |
No |
[] |
See CLOSED-CAPTIONS attribute in EXT-X-STREAM-INF
|
currentRenditions |
object ({audio: number, video: number, subtitles: number, closedCaptions: number}) |
No |
{} |
A hash object that contains array indices that points to the chosen Rendition for each type |
Property |
Type |
Required |
Default |
Description |
type |
string |
Yes |
N/A |
See TYPE attribute in EXT-X-MEDIA
|
uri |
string |
No |
undefined |
See URI attribute in EXT-X-MEDIA
|
groupId |
string |
Yes |
N/A |
See GROUP-ID attribute in EXT-X-MEDIA
|
language |
string |
No |
undefined |
See LANGUAGE attribute in EXT-X-MEDIA
|
assocLanguage |
string |
No |
undefined |
See ASSOC-LANGUAGE attribute in EXT-X-MEDIA
|
name |
string |
Yes |
N/A |
See NAME attribute in EXT-X-MEDIA
|
isDefault |
boolean |
No |
false |
See DEFAULT attribute in EXT-X-MEDIA
|
autoselect |
boolean |
No |
false |
See AUTOSELECT attribute in EXT-X-MEDIA
|
forced |
boolean |
No |
false |
See FORCED attribute in EXT-X-MEDIA
|
instreamId |
string |
No |
undefined |
See INSTREAM-ID attribute in EXT-X-MEDIA
|
characteristics |
string |
No |
undefined |
See CHARACTERISTICS attribute in EXT-X-MEDIA
|
channels |
string |
No |
undefined |
See CHANNELS attribute in EXT-X-MEDIA
|
MediaPlaylist
(extends Playlist
)
Property |
Type |
Required |
Default |
Description |
targetDuration |
number |
Yes |
N/A |
See EXT-X-TARGETDURATION
|
mediaSequenceBase |
number |
No |
0 |
See EXT-X-MEDIA-SEQUENCE
|
discontinuitySequenceBase |
number |
No |
0 |
See EXT-X-DISCONTINUITY-SEQUENCE
|
endlist |
boolean |
No |
false |
See EXT-X-ENDLIST
|
playlistType |
string |
No |
undefined |
See EXT-X-PLAYLIST-TYPE
|
isIFrame |
boolean |
No |
undefined |
See EXT-X-I-FRAMES-ONLY
|
segments |
[Segment ] |
No |
[] |
A list of available segments |
prefetchSegments |
[PrefetchSegment ] |
No |
[] |
A list of available prefetch segments |
lowLatencyCompatibility |
object ({canBlockReload: boolean, canSkipUntil: number, holdBack: number, partHoldBack: number}) |
No |
undefined |
See CAN-BLOCK-RELOAD , CAN-SKIP-UNTIL , HOLD-BACK , and PART-HOLD-BACK attributes in EXT-X-SERVER-CONTROL
|
partTargetDuration |
number |
No* |
undefined |
*Required if the playlist contains one or more EXT-X-PART tags. See EXT-X-PART-INF
|
renditionReports |
[RenditionReport ] |
No |
[] |
Update status of the associated renditions |
skip |
number |
No |
0 |
See EXT-X-SKIP
|
Property |
Type |
Required |
Default |
Description |
uri |
string |
Yes* |
N/A |
URI of the media segment. *Not required if the segment contains EXT-X-PRELOAD-HINT tag |
duration |
number |
Yes* |
N/A |
See EXTINF *Not required if the segment contains EXT-X-PRELOAD-HINT tag |
title |
string |
No |
undefined |
See EXTINF
|
byterange |
object ({length: number, offset: number}) |
No |
undefined |
See EXT-X-BYTERANGE
|
discontinuity |
boolean |
No |
undefined |
See EXT-X-DISCONTINUITY
|
mediaSequenceNumber |
number |
No |
0 |
See the description about 'Media Sequence Number' in 3. Media Segments
|
discontinuitySequence |
number |
No |
0 |
See the description about 'Discontinuity Sequence Number' in 6.2.1. General Server Responsibilities
|
key |
Key |
No |
undefined |
See EXT-X-KEY
|
map |
MediaInitializationSection |
No |
undefined |
See EXT-X-MAP
|
programDateTime |
Date |
No |
undefined |
See EXT-X-PROGRAM-DATE-TIME
|
dateRange |
DateRange |
No |
undefined |
See EXT-X-DATERANGE
|
markers |
[SpliceInfo ] |
No |
[] |
SCTE-35 messages associated with this segment |
parts |
[PartialSegment ] |
No |
[] |
Partial Segments that constitute this segment |
gap |
boolean |
No |
undefined |
See EXT-X-GAP
|
PartialSegment
(extends Data
)
Property |
Type |
Required |
Default |
Description |
hint |
boolean |
No |
false |
true indicates a hinted resource (TYPE=PART ) See EXT-X-PRELOAD-HINT
|
uri |
string |
Yes |
N/A |
See URI attribute in EXT-X-PART
|
duration |
number |
Yes* |
N/A |
See DURATION attribute in EXT-X-PART *Not required if hint is true
|
independent |
boolean |
No |
undefined |
See INDEPENDENT attribute in EXT-X-PART
|
byterange |
object ({length: number, offset: number}) |
No |
undefined |
See BYTERANGE attribute in EXT-X-PART
|
gap |
boolean |
No |
undefined |
See GAP attribute in EXT-X-PART
|
PrefetchSegment
(extends Data
)
Property |
Type |
Required |
Default |
Description |
method |
string |
Yes |
N/A |
See METHOD attribute in EXT-X-KEY
|
uri |
string |
No |
undefined |
See URI attribute in EXT-X-KEY
|
iv |
ArrayBuffer (length=16) |
No |
undefined |
See IV attribute in EXT-X-KEY
|
format |
string |
No |
undefined |
See KEYFORMAT attribute in EXT-X-KEY
|
formatVersion |
string |
No |
undefined |
See KEYFORMATVERSIONS attribute in EXT-X-KEY
|
MediaInitializationSection
Property |
Type |
Required |
Default |
Description |
hint |
boolean |
No |
false |
true indicates a hinted resource (TYPE=MAP ) See EXT-X-PRELOAD-HINT
|
uri |
string |
Yes |
N/A |
See URI attribute in EXT-X-MAP
|
byterange |
object ({length: number, offset: number}) |
No |
undefined |
See BYTERANGE attribute in EXT-X-MAP
|
Property |
Type |
Required |
Default |
Description |
id |
string |
Yes |
N/A |
See ID attribute in EXT-X-DATERANGE
|
classId |
string |
No |
undefined |
See CLASS attribute in EXT-X-DATERANGE
|
start |
Date |
No |
undefined |
See START-DATE attribute in EXT-X-DATERANGE
|
end |
Date |
No |
undefined |
See END-DATE attribute in EXT-X-DATERANGE
|
duration |
number |
No |
undefined |
See DURATION attribute in EXT-X-DATERANGE
|
plannedDuration |
number |
No |
undefined |
See PLANNED-DURATION attribute in EXT-X-DATERANGE
|
endOnNext |
boolean |
No |
undefined |
See END-ON-NEXT attribute in EXT-X-DATERANGE
|
attributes |
object |
No |
{} |
A hash object that holds SCTE35 attributes and user defined attributes. See SCTE35-* and X- attributes in EXT-X-DATERANGE
|
Only EXT-X-CUE-OUT
and EXT-X-CUE-IN
tags are supported. Other SCTE-35-related tags are stored as raw (string) values.
Property |
Type |
Required |
Default |
Description |
type |
string |
Yes |
N/A |
{'OUT', 'IN', 'RAW'} |
duration |
number |
No |
undefined |
Required if the type is 'OUT' |
tagName |
string |
No |
undefined |
Holds the tag name if any unsupported tag are found. Required if the type is 'RAW' |
value |
string |
No |
undefined |
Holds a raw (string) value for the unsupported tag. |